
Analytics transforms data into answers –
We help our customers get
the answers they're looking for.
And we do it quicker, easier, cheaper, and more precisely than they could on their own.

Game Developer
Distribution Network
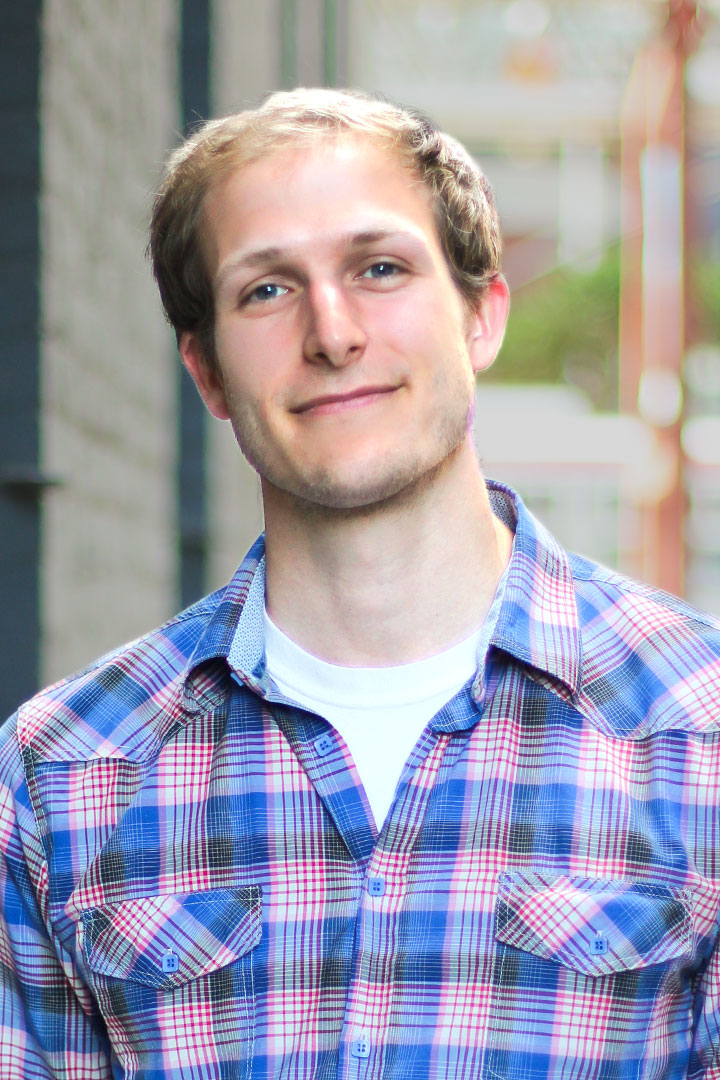
With Keen IO, I don't even have to think about how it works – I just know that it does work, and it will work.
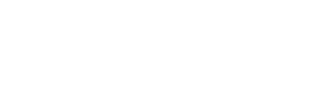
Mobile Developer Testing
& Engagement Platform
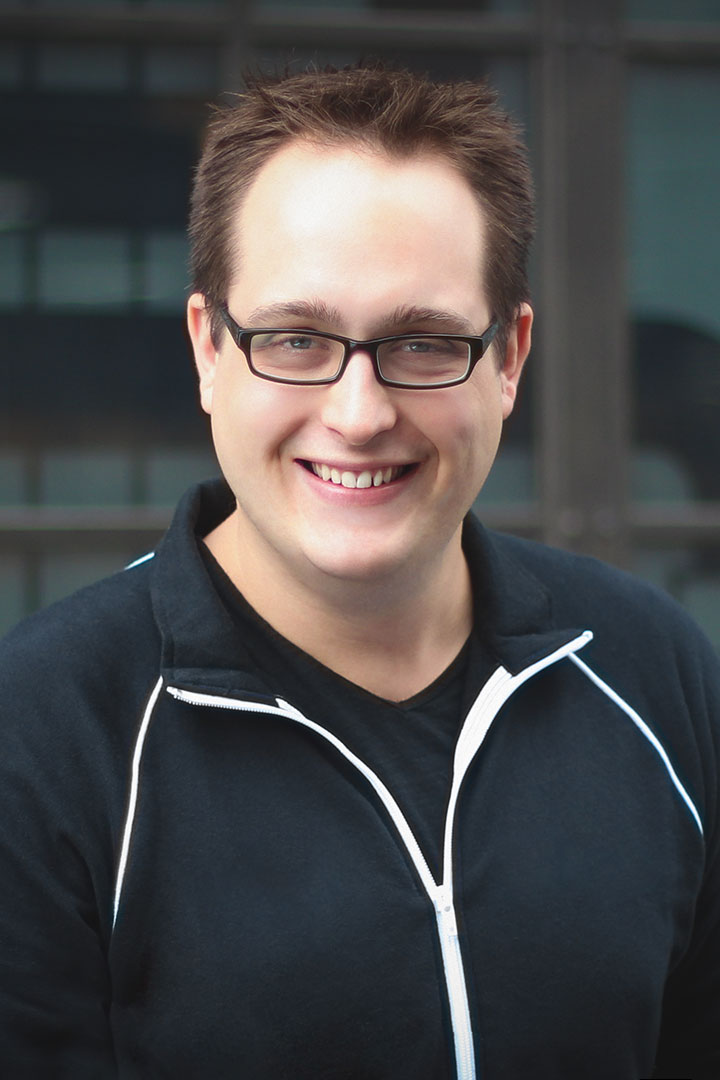
We have a wide infrastructure, and Keen IO lets us naturally and easily integrate across languages and services, tying together separate systems.
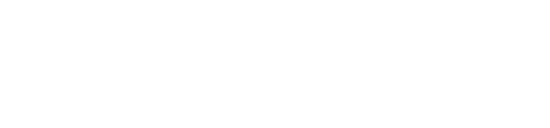
SaaS Tools for
Online Lead Generation
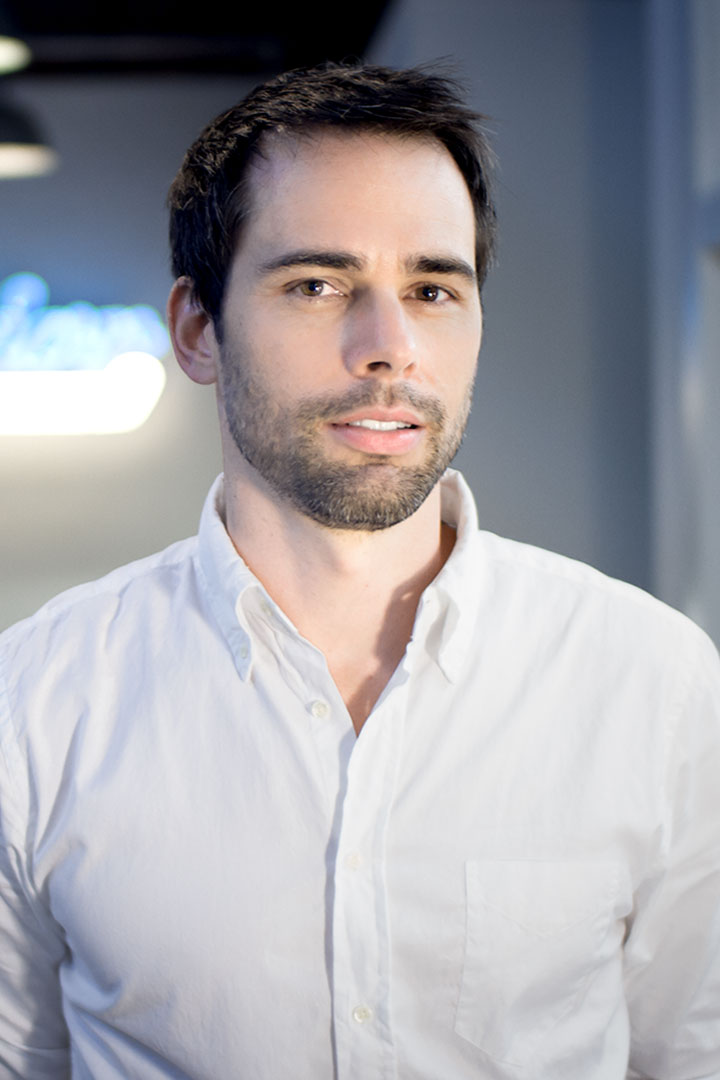
It's really easy to get up and running with Keen IO – any time you can ask questions or give feedback directly to the developers working on it, it's the best possible solution.
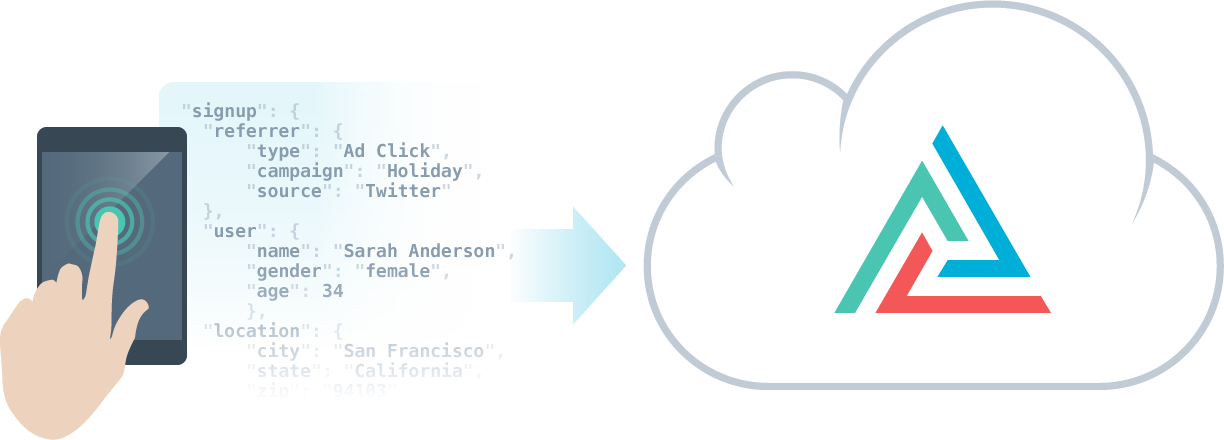
Send all your data – any event, from any source, and as much as you want.
Keen IO was built to collect and store huge amounts of event data – those constant little interactions that happen all day, every day, in your apps. You send ‘em, and we store ‘em in our cloud database, so you can play around with them whenever you’d like.
Collect data from any source
If it connects to the internet, you can use Keen IO to grab data from it: Websites, web apps, servers, mobile devices, cars, smartwatches, electronic kangaroo tracking collars – it's all good!
- Data collection is quick and painless. Use our super-simple REST API, our ready-made SDKs, or one of the, like, 8 other tools we've built.
Grab exactly the data you need
Event data can be anything – signups, upgrades, impressions, purchases, errors, shares. The arbitrary JSON format we use makes it easy to grab exactly the data you're looking for, with all of the custom properties you've been dreaming of.
- Event data is Big Data. That's why we build a massively scalable, super-resilient event data backend. Send us your worst. We can handle it.
import io.keen.client.net.Client var prjSettings = new ProjectSettingsProvider("YourProjectID", writeKey: "YourWriteKey"); var keenClient = new KeenClient(prjSettings); // Publish an event! var aPurchase = new { item = "norwegian ridgeback dragon", category = "magical animals", username = "Hagrid", price = 7013, payment_type = "dubloons" }; keenClient.AddEvent("purchases", aPurchase);
import io.keen.client.scala.Client val client = new Client( projectId = "projectId", writeKey = Some("writeKey") ) // Publish an event! client.addEvent( collection = "collectionNameHere", event = """{ price: 50.00, user: { id: "020939382", age: 28, gender: "M" }, artist: { id: "19039", name: "Meshuggah" }, venue: { id: "A93DJ", name: "Slim's", city: "San Francisco", state: "California" } }""" )
// Register your client. [KeenClient sharedClientWithProjectId:@"projectId" andWriteKey:@"writeKey" andReadKey:nil]; // Build your event properties as a dictionary. NSDictionary *ticketPurchase = @{ @"price" : @(50.00), @"user" : @{ @"id" : @"020939382", @"age" : @(28), @"gender" : @"M" }, @"artist" : @{ @"id" : @"19039", @"name" : @"Meshuggah" }, @"venue" : @{ @"id" : @"A93DJ", @"name" : @"Slim's", @"city" : @"San Francisco", @"state" : @"California" } }; // Add your event to the "ticket_purchases" collection. [[KeenClient sharedClient] addEvent:ticketPurchase toCollection:@"ticket_purchases"]; // Send us your tracked events. [[KeenClient sharedClient] uploadWithFinishedBlock:nil];
// Register your client. KeenClient.initialize(getApplicationContext(), "projectId", "writeKey", null); // Build your event properties as a Map. Map<String, Object> userData = new HashMap<String, Object>(); userData.put("id", "020939382"); userData.put("age", "28"); userData.put("gender", "M"); Map<String, Object> artistData = new HashMap<String, Object>(); artistData.put("id", "19039"); artistData.put("name", "Meshuggah"); Map<String, Object> venueData = new HashMap<String, Object>(); venueData.put("id", "A93DJ"); venueData.put("name", "Slim's"); venueData.put("city", "San Francisco"); venueData.put("state", "California"); Map<String, Object> ticketPurchase = new HashMap<String, Object>(); ticketPurchaseData.put("price", 50.00); ticketPurchaseData.put("user", userData); ticketPurchaseData.put("artist", artistData); ticketPurchaseData.put("venue", venueData); // Add your event to the "ticket_purchases" collection. KeenClient.client().addEvent("ticket_purchases", ticketPurchase); // Send us your tracked events. KeenClient.client().upload();
// Register your client $client = KeenIOClient::factory([ 'projectId' => $projectId, 'writeKey' => $writeKey ]); // Build your event properties as an associative array. $ticketPurchase = [ "price" => 50.00, "user" => [ "id" => "020939382", "age" => 28, "gender" => "M" ], "artist" => [ "id" => "19039", "name" => "Meshuggah" ], "venue" => [ "id" => "A93DJ", "name" => "Slim's", "city" => "San Francisco", "state" => "California" ] ] // Add your event to the "ticket_purchases" collection. $client->addEvent( "ticket_purchases", [ "data" => $ticketPurchase ] );
// Create your Keen Client var client = new Keen({ projectId: "your_project_id", writeKey: "your_write_key" }); // Build your event properties as a JavaScript Object. var ticketPurchase = { price: 50.00, user: { id: "020939382", age: 28, gender: "M" }, artist: { id: "19039", name: "Meshuggah" }, venue: { id: "A93DJ", name: "Slim's", city: "San Francisco", state: "California" } } // Add your event to the "ticket_purchases" collection. client.addEvent("ticket_purchases", ticketPurchase);
# Initialize the Keen Client. keen = Keen::Client.new(:project_id => "project_id", :write_key => "write_key") # Build your event properties as a hash. ticket_purchase = { :price => 50.00, :user => { :id => "020939382", :age => 28, :gender => "M" }, :artist => { :id => "19039", :name => "Meshuggah" }, :venue => { :id => "A93DJ", :name => "Slim's", :city => "San Francisco", :state => "California" } } # Add your event to the "ticket_purchases" collection. keen.add_event("ticket_purchases", ticket_purchase)
# Initialize the Keen Client. keen = keen.client.KeenClient("project_id", write_key="write_key") # Build your event properties as a dictionary. ticket_purchase = { "price" : 50.00, "user": { "id": "020939382", "age": 28, "gender": "M" }, "artist": { "id": "19039", "name": "Meshuggah" }, "venue": { "id": "A93DJ", "name": "Slim's", "city": "San Francisco", "state": "California" } } # Add your event to the "ticket_purchases" collection. keen.add_event("ticket_purchases", ticket_purchase)
// Register your client. KeenClient.initialize("projectId", "writeKey", null); // Build your event properties as a Map. Map<String, Object> userData = new HashMap<String, Object>(); userData.put("id", "020939382"); userData.put("age", "28"); userData.put("gender", "M"); Map<String, Object> artistData = new HashMap<String, Object>(); artistData.put("id", "19039"); artistData.put("name", "Meshuggah"); Map<String, Object> venueData = new HashMap<String, Object>(); venueData.put("id", "A93DJ"); venueData.put("name", "Slim's"); venueData.put("city", "San Francisco"); venueData.put("state", "California"); Map<String, Object> ticketPurchase = new HashMap<String, Object>(); ticketPurchaseData.put("price", 50.00); ticketPurchaseData.put("user", userData); ticketPurchaseData.put("artist", artistData); ticketPurchaseData.put("venue", venueData); // Add your event to the "ticket_purchases" collection. KeenClient.client().addEvent("ticket_purchases", ticketPurchase); // Send us your tracked events. KeenClient.client().upload();
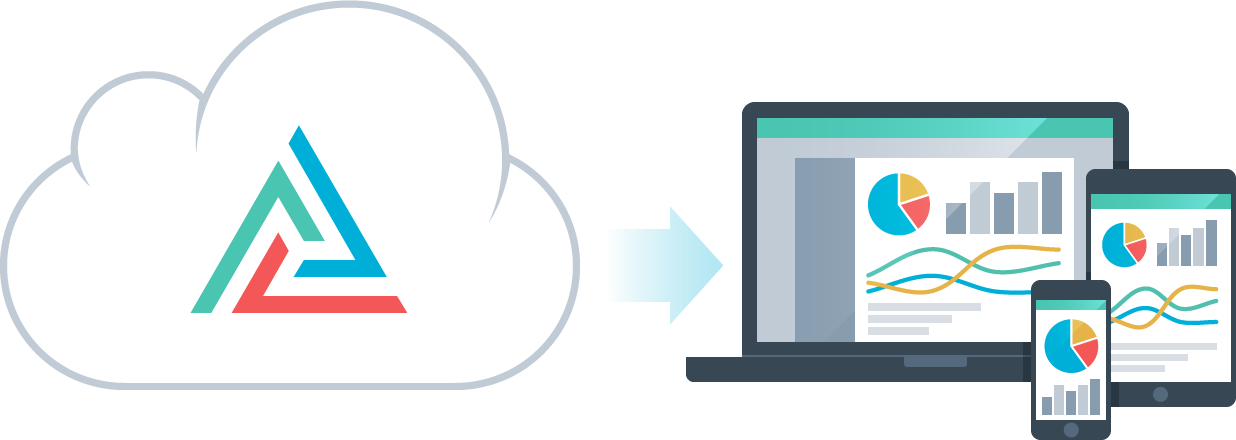
Analyze, visualize, find answers.
Once you've sent us some events, that's when the real fun can begin. Our powerful analysis API lets you perform any sort of query you need today – and any you might think of in the future. And our visualization tools help you chart that data and transform it into answers.
Ask the questions you want to ask.
Unlike other analytics services, which usually only offer pre-defined reports, Keen IO gives you the tools to measure exactly what you want. Any property, any query, any way you'd like it.
- Our intuitive query API has a ton of built-in resources that allow you to do all the analytics must-haves: Counts and sums, mins and maxes, averages, all that jazz.
- Create queries on the fly and get responses back in real-time , no matter the size of your dataset. Because you shouldn't have to wait for answers.
- Combine elements of our analysis APIs to build the dashboard of your dreams. Segment data by timeframe, interval, and groups. Create funnels and cohort analyses. Measure conversion and engagement. Make magic happen.
Make answers tangible with embeddable charts and metrics
We make it easy to draw metrics, line charts, pie charts, and funnels with our JavaScript SDK, but you can also use your Keen IO data with any charting library to customize your look and functionality as you see fit.
var series = new Keen.Query("count", { eventCollection: "ticket_purchases", timeframe: "previous_7_days", interval: "daily" }); client.draw(series, document.getElementById("chartEl"), { chartType: "linechart", label: "Tickets", title: "Ticket Purchases By Day" });
var funnel = new Keen.Query("funnel", { steps: [ { eventCollection: "download app", actorProperty: "user.id" }, { eventCollection: "create account", actorProperty: "user.id" }, { eventCollection: "ticket purchase", actorProperty: "user.id" }, ], timeframe: "this_6_months" }); client.draw(funnel, document.getElementById("chartEl"), { title: "User Life Cycle Funnel", chartType: "columnchart", chartOptions: { backgroundColor: "transparent", bar: { groupWidth: '90%' } }, });
$7.3k
Yesterday's Total Revenue
var metric = new Keen.Metric("ticket_purchases", { analysisType: "sum", targetProperty: "price", timeframe: "yesterday" }); client.draw(metric, document.getElementById("chartEl"), { chartType: "metric", label: "Yesterday's Total Revenue" });
var data = new Keen.Query("count", { eventCollection: "ticket_purchases", timeframe: "previous_7_days", groupBy: "venue.state" }); client.draw(data, document.getElementById("chartEl"), { chartType: "piechart", title: "Ticket Purchases by State", colors: [ "#00afd7", "#49c5b1", "#e6b449", "#f35757"] });
Share whatever you want, with whoever you want – it's your data, after all!
Because your analytics are available by API, what you do with them is entirely up to you. Share them with your team, your customers, your partners, your investors, whoever! Spot trends. Show value. Test. Debug. Make data actionable.
White-labeled analytics for your customers
Keen IO was built for white-labeled analytics – you can share data on your website, your customer's website, your mobile app, or wherever else you might need it.
- Share full data for internal use, or securely filter it out into custom dashboards for your customers.
Extract data painlessly
We made it simple to extract full-resolution events by API or .CSV download, any time you need them. It's your data – you should always have access to it!